This article guides you through deploying a simple machine learning model for regression using Streamlit, a platform designed to streamline the deployment of machine learning systems as web services.
Overview of the Deployment Process
In this tutorial, we’ll focus on getting a machine learning model up and running in the cloud within minutes. We’ll create and deploy a straightforward linear regression model to predict house prices based on just one attribute: house size in square feet.
Starting Point – The Code
Here’s the code we will use:
import streamlit as st
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
import plotly.express as px
# Generate synthetic data for training the model
def generate_house_data(n_samples=100):
np.random.seed(42)
size = np.random.normal(1500, 500, n_samples)
price = size * 100 + np.random.normal(0, 10000, n_samples)
return pd.DataFrame({'size_sqft': size, 'price': price})
# Train the linear regression model
def train_model():
df = generate_house_data()
X = df[['size_sqft']]
y = df['price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
return model
# Streamlit User Interface
def main():
st.title('🏠 Simple House Pricing Predictor')
st.write('Enter house size to predict sale price')
model = train_model()
size = st.number_input('House size (square feet)', min_value=500, max_value=5000, value=1500)
if st.button('Predict price'):
prediction = model.predict([[size]])
st.success(f'Estimated price: ${prediction[0]:,.2f}')
# Visualization
df = generate_house_data()
fig = px.scatter(df, x='size_sqft', y='price', title='Size vs Price Relationship')
fig.add_scatter(x=[size], y=[prediction[0]], mode='markers', marker=dict(size=15, color='red'), name='Prediction')
st.plotly_chart(fig)
if __name__ == '__main__':
main()
Key Aspects of the Code
- The
main()
function initializes the Streamlit interface for user interaction. - It trains the model and provides an input field for users to enter the house size and get a price prediction.
- Upon clicking the button, Streamlit processes the prediction and displays the results alongside a visualization.
Deployment Process
- Save Code: Copy the above code into a
.py
file and set up a GitHub repository including arequirements.txt
file with:
streamlit==1.29.0
pandas==2.1.4
numpy==1.26.2
scikit-learn==1.3.2
plotly==5.18.0
- Deploy on Streamlit Cloud: Create an account on Streamlit Cloud, click on “Create App”, and select the option to deploy from GitHub. Provide your repository URL, branch, and file information, then click deploy.
- Access your App: Your deployed model will now be accessible through the provided URL.
Conclusion
Congratulations! You’ve successfully deployed a machine learning model using Streamlit.
Generated Images for Explanation
Here is an image of a tutorial layout showcasing the deployment of a simple machine learning model using Streamlit. It includes elements such as code snippets for Python, an image of a web interface with ML predictions, and illustrations of data generation and model training.
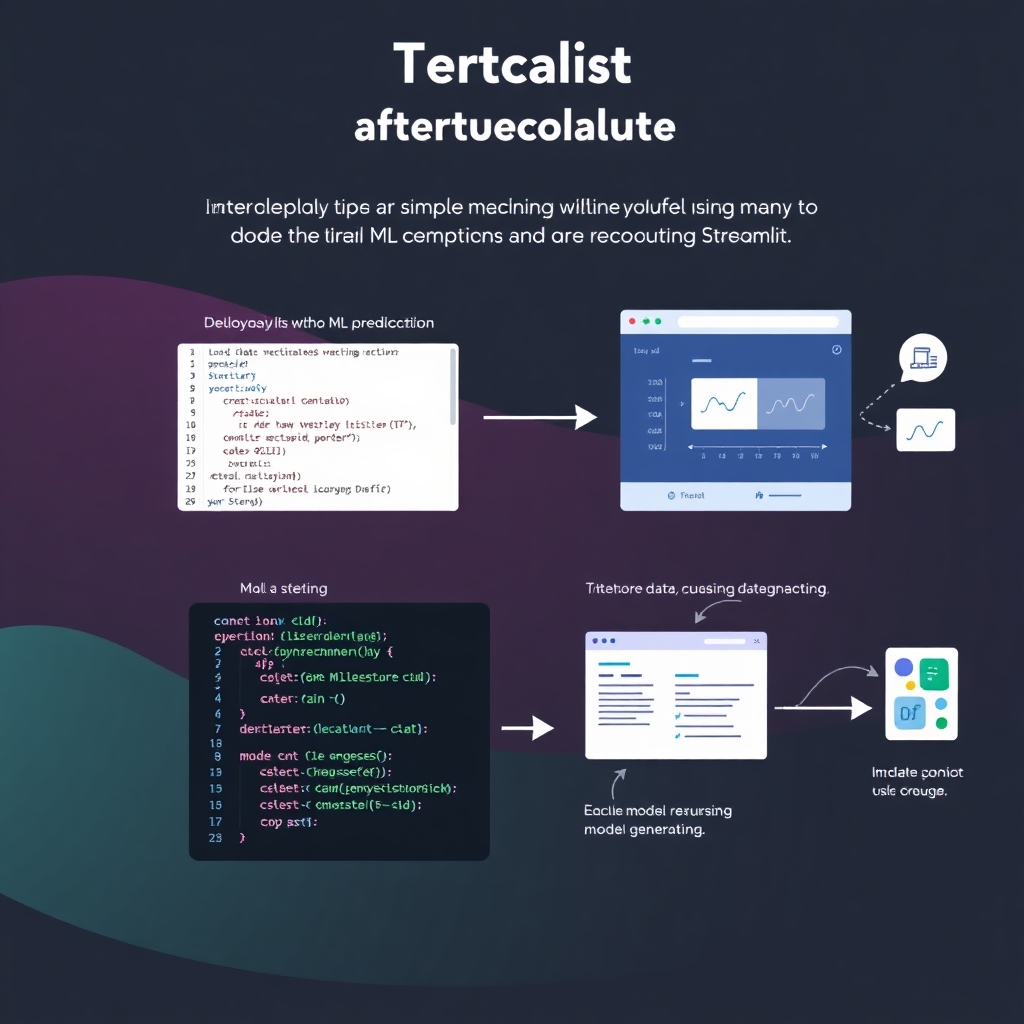
Here is an infographic illustrating the process of deploying a machine learning model on Streamlit. It includes steps such as writing code in Python, creating a GitHub repository, uploading a requirements.txt file, and the deployment interface of Streamlit Cloud.
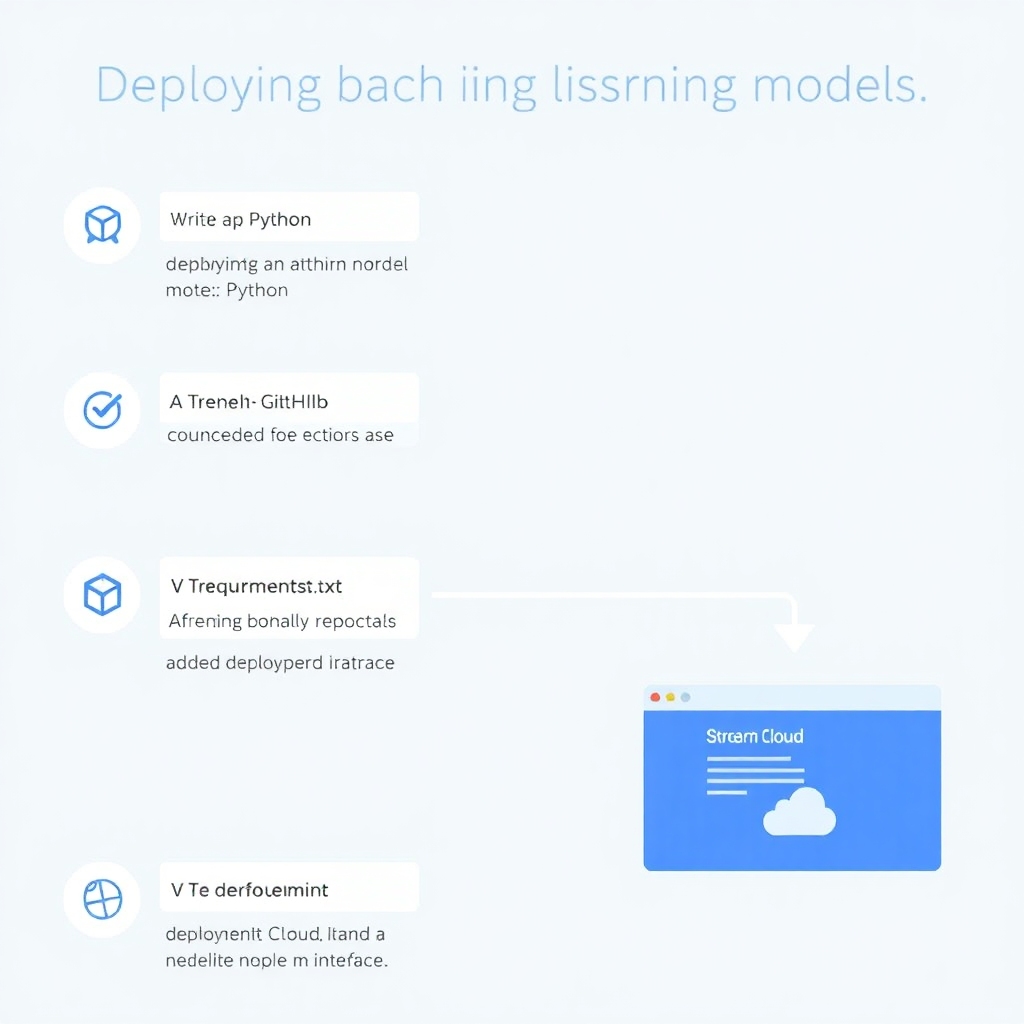
Feel free to reach out if you have any more questions or need further assistance!