I’ve worked on various projects in computer vision and machine learning, from object recognition to face recognition systems, including an open-source Python library for face recognition that’s among the top 10 machine learning libraries on GitHub. This popularity means I frequently receive inquiries from newcomers to Python and computer vision.
One technical issue tends to confuse users more than any other: it’s not about complex theories or pricey GPUs. Surprisingly, it’s the common problem of loading images into memory in the wrong orientation, often sideways, without realizing it. This creates difficulties for computers when detecting objects or recognizing faces in these incorrectly oriented images.
How Digital Cameras Work with Image Orientation
When you take a photograph, the camera detects the tilt of the device. This allows for proper viewing in other applications later. However, the camera does not actually modify the image data when saving it. Instead, it saves the pixel data in a consistent order, regardless of how the camera is held.
The key lies in the metadata; alongside the image, cameras store various details, including the rotation angle required for correct display. It’s up to the image viewer to interpret and adjust the display based on this Exif (Exchangeable Image File Format) data.
Here’s a look at the Exif metadata from an example JPEG image:
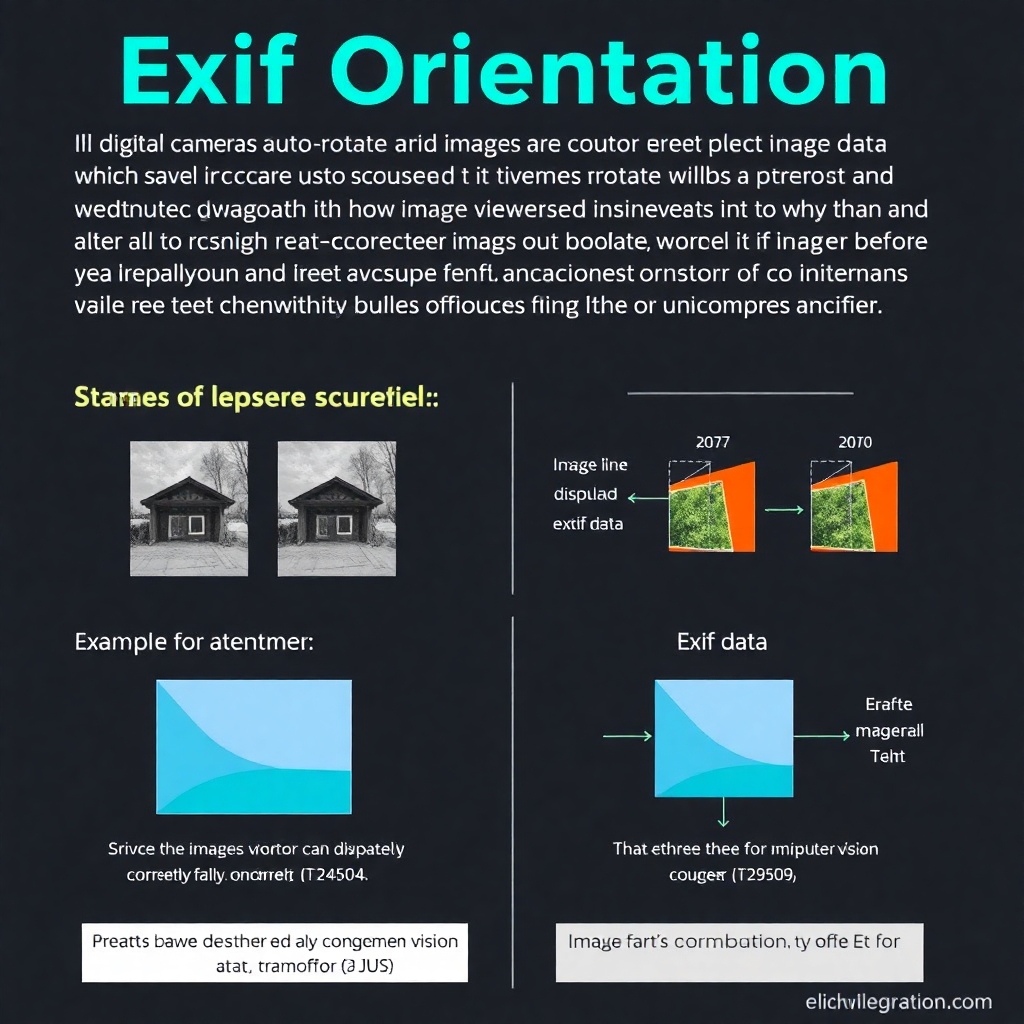
Why This Causes Trouble in Python Computer Vision Applications
Exif metadata is not inherently part of the JPEG format; it was added later for compatibility with older viewers. Many Python libraries, such as NumPy, SciPy, TensorFlow, and Keras, treat images strictly as arrays and do not consider consumer-level concerns like automatic rotation. As a result, they often load the original, unmodified image data, leading to errors in detection when the input is incorrectly oriented.
This issue isn’t solely a beginner’s mistake; even robust APIs like Google Vision have faced similar problems. For instance, in tests, Google Vision sometimes fails to appropriately handle portrait-oriented images captured by standard smartphones.
When input with proper rotation, images return more accurate labels and confidence scores, highlighting the significant impact of orientation:
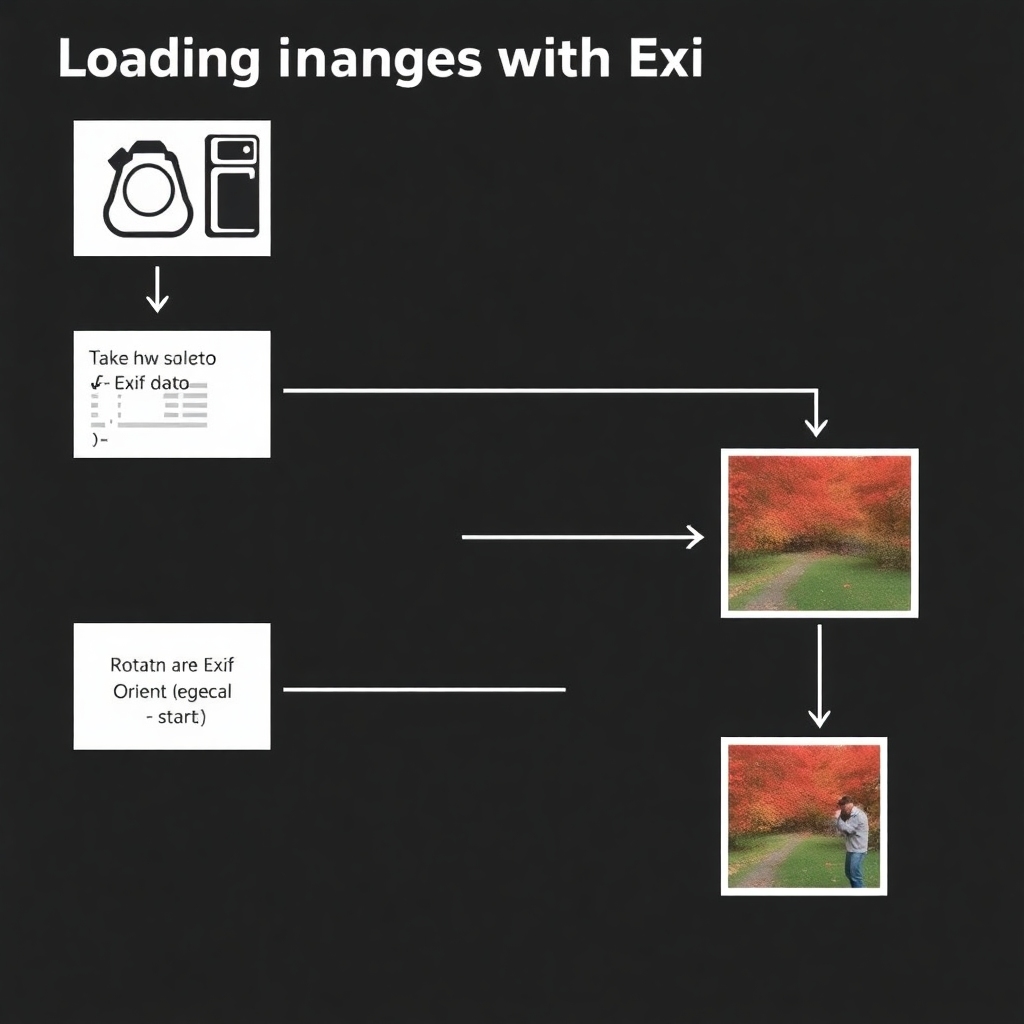
Resolving the Issue
To tackle this problem, ensure that you check for Exif Orientation metadata every time you load images in your Python programs. While it’s straightforward, finding reliable code examples for proper rotation can be challenging.
Here’s a brief example of how to load an image correctly using a library designed for that purpose:
import matplotlib.pyplot as plt
import image_to_numpy
# Load your image file
img = image_to_numpy.load_image_file("my_file.jpg")
# Display the image
plt.imshow(img)
plt.show()
For convenience, this functionality is available through the library image_to_numpy
, which can be installed via pip:
pip3 install image_to_numpy
Check the README file for further details. Enjoy coding with proper image handling!